GameMaker: Studio has a number of constants and functions related to the keyboard and how it can be used to make things happen in your games.
GameMaker is an easy to use card based development system which allows you to create simple adventure games with graphics, buttons, and text. Just draw the pictures, type some text, click a few buttons, choose some options. And an adventure game is completed. You are not required to learn a programming language.
When dealing with the keyboard in GameMaker: Studio you have a variety of functions that can be used to recognise different keyboard states like pressed or released. There are also some that store all the keypresses as a string or that can tell you what the last key pressed was, as well as others that allow you to clear the keyboard state completely.
NOTE: These functions are designed for Windows/Mac/Ubuntu desktop platforms only. You may find some of the in-built variables and constants aren't valid on other platforms and many of the functions won't work on mobiles.
Now, each key is normally defined by a number, called the ascii code, and you can directly input this number into these functions and they will work fine... But, as it's a bit difficult to remember so many numbers and the relationship that they have with your keyboard, GameMaker: Studio has a series of constants for the most used keyboard special keys and a special function ord() to return the number from ordinary typed characters (either letters or numbers).
The following is a small example of how to use ord():
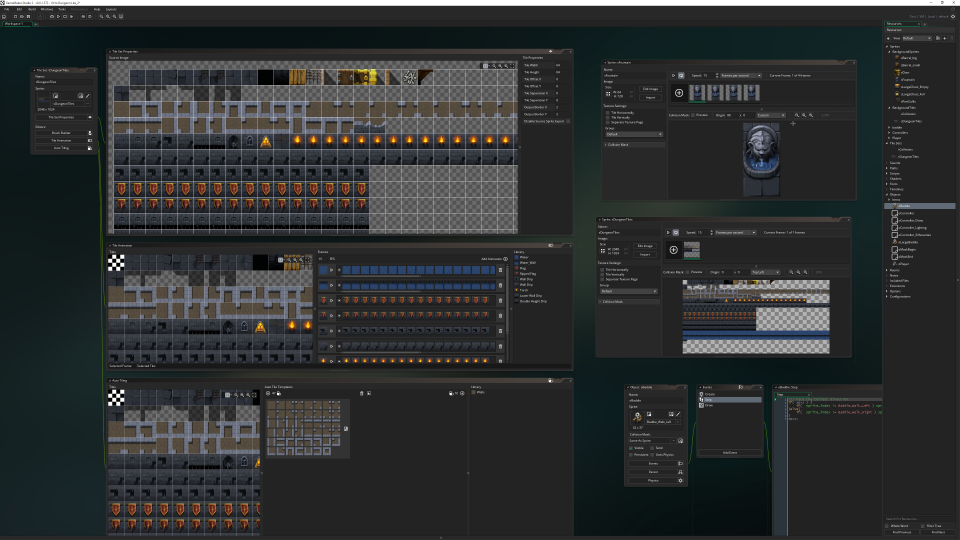
if keyboard_check(ord('A'))
{
hspeed = -5;
}
So, the above will check the 'A' key and if it's being pressed then it'll set the horizontal speed of the object to -5. Note, that the 'A' is a capital 'A', and that when using ord() the keyboard key to check must always be written in quotes and as a capital. Now, what if you want to use the arrow keys? Or if you want to modify an action using the 'shift' key? Well, for that GameMaker: Studio has a series of vk_ constants (vk_ stands for virtual keyboard) that you can use in place of ord or the ascii code.
Here is a complete list of the vk_ constants:
Constant | Description |
---|---|
vk_nokey | keycode representing that no key is pressed |
vk_anykey | keycode representing that any key is pressed |
vk_left | keycode for left arrow key |
vk_right | keycode for right arrow key |
vk_up | keycode for up arrow key |
vk_down | keycode for down arrow key |
vk_enter | enter key |
vk_escape | escape key |
vk_space | space key |
vk_shift | either of the shift keys |
vk_control | either of the control keys |
vk_alt | alt key |
vk_backspace | backspace key |
vk_tab | tab key |
vk_home | home key |
vk_end | end key |
vk_delete | delete key |
vk_insert | insert key |
vk_pageup | pageup key |
vk_pagedown | pagedown key |
vk_pause | pause/break key |
vk_printscreen | printscreen/sysrq key |
vk_f1 ... vk_f12 | keycode for the function keys F1 to F12 |
vk_numpad0 ... vk_numpad9 | number keys on the numeric keypad |
vk_multiply | multiply key on the numeric keypad |
vk_divide | divide key on the numeric keypad |
vk_add | key on the numeric keypad |
vk_subtract | subtract key on the numeric keypad |
vk_decimal | decimal dot keys on the numeric keypad |
The following constants can only be used with keyboard_check_direct():
Constant | Description |
---|---|
vk_lshift | left shift key |
vk_lcontrol | left control key |
vk_lalt | left alt key |
vk_rshift | right shift key |
vk_rcontrol | right control key |
vk_ralt | right alt key |
The following is a small example of how to use the vk_ constants:
if keyboard_check_pressed(vk_tab)
{
instance_create(x,y,obj_Menu);
}
Game Maker Studio 2 Download Free
The above code will detect if the 'Tab' key is pressed and create an instance of object 'obj_Menu' if it is.
For information on the available GameMaker: Studio keyboard functions, please see the following sections of the manual:
- io_clear
- keyboard_check
- keyboard_check_pressed
- keyboard_check_released
- keyboard_check_direct
- keyboard_clear
- keyboard_key_press
- keyboard_key_release
- keyboard_key
- keyboard_lastkey
- keyboard_lastchar
- keyboard_string
- keyboard_set_map
- keyboard_get_map
- keyboard_unset_map
- keyboard_get_numlock
- keyboard_set_numlock